Dependency Injection (DI) is one of the fundamental characteristics enabling ASP.NET Core applications to be so maintainable. The 2022 Stack Overflow Developer Survey ranked maintainability and testability among the top concerns for developers selecting frameworks, underlining the need of Dependency Injection in building modular and flexible applications. Though the idea is easier than you might think, beginners may find it frightening.
Contact Here For SEO Service or Guest Post: http://wa.me/+923148487754
Dependency Injection is mostly about designing your application such that its components are only loosely coupled. It lets programmers modify, expand, or test individual components of an application without compromising the others. This guide will walk you through what DI is, why it is valuable, and how to apply it in ASP.NET Core projects successfully.
What is Dependency Injection?
Dependency Injection is a software design technique whereby an external source supplies the dependencies of an object instead of the object itself creating them. Though the objective is simpleβto make components independent of one another so they are easier to manage, test, and scaleβthis could sound abstract. In actual use, DI serves as a link between several components of your application in a methodical, reusable fashion.
Benefits of Dependency Injection
- Reduces Tight Coupling: Components can interact without directly depending on one another, so lowering tight coupling. When one component changes, this lowers the possibility of breaking the application.
- Improves Testability: Mock dependencies can be introduced during testing, enhancing the dependability and simplicity of unit tests and their writing ease.
- Enhances Maintainability: As your application gets more complicated, code gets more modular and refactorings and extensions become simpler.
- Promotes Reusability: Encouragement of reusability helps to save development time by allowing components to be used across several areas of the application or even in other projects.
- Centralized Configuration: With DI, all dependency configurations are handled in one location, so facilitating modification or expansion as needs develop.
For instance, an email service included into your application. You might hardcode the email provider inside the service without DI, which would make switching providers challenging or independently testing the service impossible. DI allows you to inject any email provider you choose, enhancing future-proofing of your codebase and flexibility.
Dependency Injection in ASP.NET Core
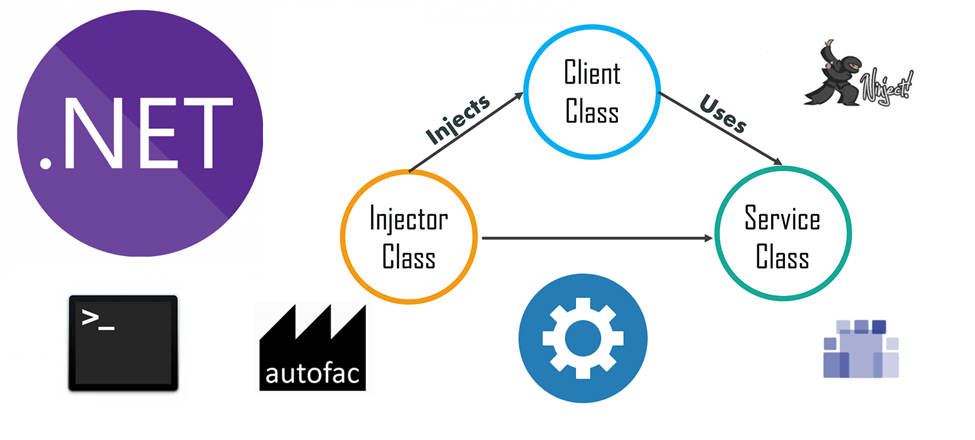
Dependency Injection is supported natively by ASP.NET Core across the IServiceCollection interface. This streamlines the management of dependencies in your application, so removing most of the need for outside libraries.
1. Service Lifetimes
You may specify lifetime for registered services in ASP.NET Core. These ascertain the duration of availability of a given instance of the service. Managing resources wisely and preventing memory leaks depend on an awareness of lifetime.
- Singleton: One single instance generated and shared over the lifetime of the application.
- Example: services.AddSingleton();
- Use case: Services that maintain global state, like configuration settings or caching mechanisms.
- Scoped: Every HTTP request generates a fresh instance under scoped.
- Example: services.AddScoped();
- Use case: Services that handle request-specific data, like database contexts or user session data.
- Transient: Every time the service is asked for, a fresh instance is generated.
- Example: services.AddTransient();
- Use case: Lightweight services with no shared state, like utility functions or logging.
2. Registering Services
Services are registered using the ConfigureServices method in your ASP.NET Core application’s Startup.cs or Program.cs file. Hereβs how it looks:
public void ConfigureServices(IServiceCollection services) { services.AddSingleton<IMySingletonService, MySingletonService>(); services.AddScoped<IMyScopedService, MyScopedService>(); services.AddTransient<IMyTransientService, MyTransientService>(); } |
Practical Examples
Let us examine practical applications for Dependency Injection. These illustrations will help you to see how DI improves application maintainability and simplifies coding chores.
1. Including Services Into Controllers
ASP.NET Core’s controllers can ask directly for dependencies using constructor injection. For instance:
public class HomeController : Controller { private readonly IMyService _myService; public HomeController(IMyService myService) { _myService = myService; } public IActionResult Index() { var data = _myService.GetData(); return View(data); } } |
This method guarantees that the Home Controller will not have to know how IMyService is run, hence replacement or mocking the service during testing will be simpler.
2. Using DI in Middleware
DI can also be used in Middleware components. For instance:
public class CustomMiddleware { private readonly RequestDelegate _next; private readonly IMyService _myService; public CustomMiddleware(RequestDelegate next, IMyService myService) { _next = next; _myService = myService; } public async Task InvokeAsync(HttpContext context) { _myService.DoSomething(); await _next(context); } } |
This setup enables middleware to focus on specific tasks while reusing existing services.
3. DI in Razor Pages
Razor Pages also support constructor injection. For example:
public class IndexModel : PageModel { private readonly IMyService _myService; public IndexModel(IMyService myService) { _myService = myService; } public void OnGet() { var result = _myService.GetData(); } } |
Razor Pages stay neat and targeted by following this pattern; dependencies are managed outside.
Advanced Techniques
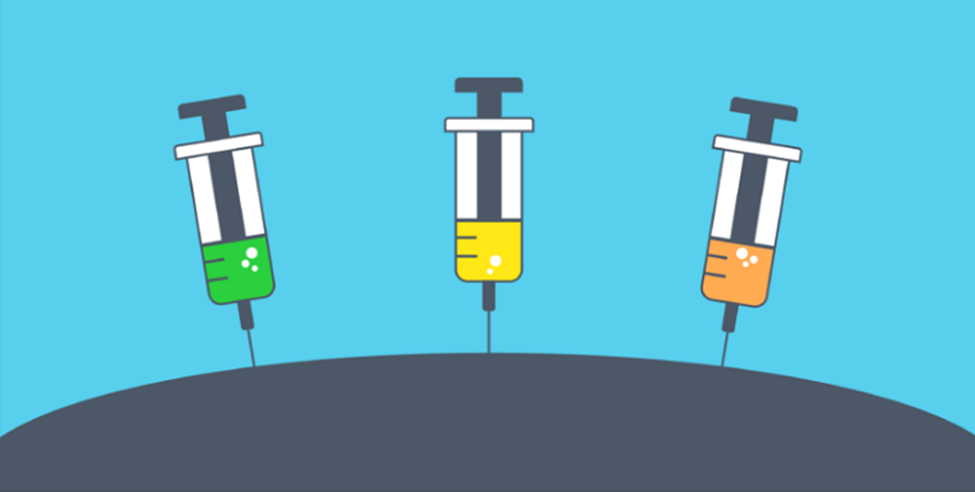
Although Dependency Injection (DI) in ASP.NET Core provides flexibility, in some cases developers may have to use more sophisticated methods to handle particular problems or improve capability.
1. Using Third-Party DI Frameworks
- Although built-in DI in ASP.NET Core is strong, some developers choose third-party frameworks like Autofac for more sophisticated capabilities. To make use of Autofac:
- Install the package Autofac NuGet.
- Switch IService Collection for Autofac’s Container Builder.
- Register systems using Autofac’s syntax.
Example:
var builder = new ContainerBuilder(); builder.RegisterType<MyService>().As<IMyService>(); |
Autofac allows advanced configuration, such as registering multiple implementations of the same interface.
2. Conditional Dependency Resolution
For scenarios where the implementation of a service depends on certain conditions, you can use a factory method:
services.AddTransient<IMyService>(provider => { var config = provider.GetService<IConfiguration>(); return config[“UseAdvancedService”] == “true” ? new AdvancedService() : new BasicService(); }); |
This approach is useful for applications with dynamic behavior based on runtime settings.
3. Managing Large-Scale Projects
Managing DI can get difficult in big applications. Here are some suggestions:
- For modular organization, use separate ServiceCollect extensions.
- Organize related services into modules tailored for particular features to maintain neat setups.
- For simpler maintenance and new developer onboarding, document dependencies.
Conclusion
Dependency Injection streamlines ASP.NET Core application architecture is injection. Decoupling elements helps to preserve scalability, testability, and maintainability. From registering services to sophisticated techniques like conditional resolution, DI gives developers great freedom.
Learning DI will help you to advance your development skills, whether you are just beginning or working on challenging projects. Effective implementation of DI helps you to design applications that are simpler to test, extend, and maintain, guaranteeing long-term success in your development projects.
Nicely explained
The examples provided are practical and relevant. A downloadable sample project would make the article even more useful.
The article is beginner-friendly. Adding a comparison between .NET technologies and other frameworks could be insightful.
The content is thorough and well-organized. Including links to further reading or tutorials would enrich the experience
A great resource for understanding .NET. A conclusion summarizing the key points would wrap it up nicely.
The content is informative and easy to read. Including diagrams of workflows or architectures would help visualize the concepts better.
A very beginner-friendly guide. Including a section on the history and evolution of .NET could give readers more context.
The explanations are straightforward and clear. Offering real-world scenarios where .NET is used would make the content more relatable.
A comprehensive overview of .NET technologies. Including industry trends or future developments would add more depth.
The examples provided are clear and practical. A video demonstration or interactive content could make it more engaging.
The article is a great starting point for learners. Including a section on how to set up the development environment would be helpful.
The content is easy to digest and well-organized. Adding real-world success stories using .NET could inspire readers.
The content covers the basics effectively. Expanding on advanced topics could benefit a wider audience.
The article is concise and straightforward. Adding references to official documentation could improve its credibility.
The article balances technical accuracy with simplicity. Including a case study would make it more relatable to practical scenarios.