Did you know that developers spend a lot of time—estimates range from 26% to 75%—debugging and fixing problems, so stressing the need for proactive testing strategies like unit testing? Early problem identification and guaranteed code performance help unit testing greatly ease this load. Learning unit testing for developers of.NET is about producing dependable, maintainable, and high-quality code, not only about spotting flaws. Using the correct tools and techniques will help you to improve software, expedite your development process, and simplify next upgrades.
This article will go over the value of unit testing, how to set up your testing environment, how to create successful tests, and even how to apply cutting-edge methods to advance your abilities.
Importance of Unit Testing
Unit testing is isolating individual, single components of your code testing. In contemporary software development, it is among the best strategies for preserving code quality. It guarantees not only functionality but also acts as documentation for expected behavior, facilitating the onboarding of new team members.
Why Unit Testing Matters?
- Catching Bugs Early: Early discovery of a bug makes fixing less expensive. Unit tests enable you to find problems long before they ever go into use during development. Industry statistics show that fixing issues during the design stage costs six times less than fixing them following implementation.
- Simplifying Refactoring: Tests serve as a safety net when you change code so that your updates do not compromise already-existing capability. This motivates ongoing development and lessens the difficulty of code refactoring.
- Improving Collaboration: Unit tests give teams a clear definition of expected behavior, which facilitates their working on the same codebase free from stepping on each other’s toes. Particularly in agile contexts, this increases team effectiveness.
- Supporting Continuous Integration (CI): CI pipelines include automated unit tests, which let you find mistakes before they become a main branch merging point. CI pipelines having strong unit tests show up to 50% less integration problems.
Real-World Impact
Strong testing cultures have been linked in real-world impact studies to lower production errors, faster development cycles, and increased user satisfaction. For example, companies who give unit testing top priority have been shown to lower production downtime by as much as 30%. Investing in unit testing helps you to improve the general development process and product quality, not only your code.
Setting Up Unit Tests in .NET

Starting with unit testing in. NET is simple thanks to the great variety of tools and frameworks available. Knowing how to arrange your surroundings will save time and enable better testing efficiency.
1. Choosing a Framework
- MSTest: MSTest is a built-in framework for .Net for those looking for a basic, native solution.
- xUnit: Often regarded as the benchmark for .NET projects, xUnit is known for its adaptability and general acceptance. It provides powerful community support and advanced capabilities including parallel test running.
- NUnit: Another often used option with a great feature set and strong community support is NUnit. For projects requiring significant parameterized testing especially, it is preferred.
2. Installing a Framework
To install a framework like xUnit in your .NET project, use the NuGet Package Manager:
Install-Package xunit |
3. Setting Up a Test Project
Establish a separate test project to maintain orderly your tests. If your primary project is named MyApp, for instance, open a new project called MyApp.Tests. Organizing tests into a separate project guarantees better division of issues.
dotnet new xunit -n MyApp.Tests |
4. Writing Your First Test
Here’s a simple example of a unit test using xUnit:
using Xunit; public class CalculatorTests { [Fact] public void Add_ShouldReturnCorrectSum() { // Arrange var calculator = new Calculator(); // Act var result = calculator.Add(2, 3); // Assert Assert.Equal(5, result); } } |
This test looks at whether the Calculator class’s Add method operates as expected. Using a clear Arrange-Act-Assert (AAA) pattern maintains the logical and simple to follow test structure.
Writing Effective Unit Tests
Writing tests involves more than just stuffing assertions into your code. Good tests are maintainable, simple, and understandable. They should be written keeping future developers in mind so that they remain valuable as the code develops.
Best Practices for Writing Tests
- Keep Tests Small and Focused: Every test should confirm just one capability. This simplifies debugging tests should something go wrong.
- Use Descriptive Names: Test names should exactly state what they are testing for. Use CalculateTotal_Returns Correct Value, for instance, rather than Test 1.
- Avoid Dependencies: Tests should run autonomously free from depending on outside systems like databases or APIs. Moq and other mocking instruments let you replicate these dependencies.
- Test Edge Cases: Test more than just the “happy path.” Think through situations like null inputs or extreme values where things might go wrong.
- Make Tests Repeatable: Every time they are administered, tests should yield the same findings. This guarantees homogeneity in many surroundings.
Using Mocking Frameworks
For isolating the unit under test, mocking frameworks like Moq are quite helpful. They let you replicate difficult dependencies without creating real instances. For example:
using Moq; using Xunit; public class OrderServiceTests { [Fact] public void PlaceOrder_ShouldCallInventoryService() { // Arrange var inventoryServiceMock = new Mock<IInventoryService>(); var orderService = new OrderService(inventoryServiceMock.Object); // Act orderService.PlaceOrder(new Order()); // Assert inventoryServiceMock.Verify(service => service.CheckStock(It.IsAny<int>()), Times.Once); } } |
This test guarantees the PlaceOrder approach calls the CheckStock method on the inventory service. By removing outside dependencies, mocking speeds and increasing dependability of your tests.
Advanced Testing Techniques
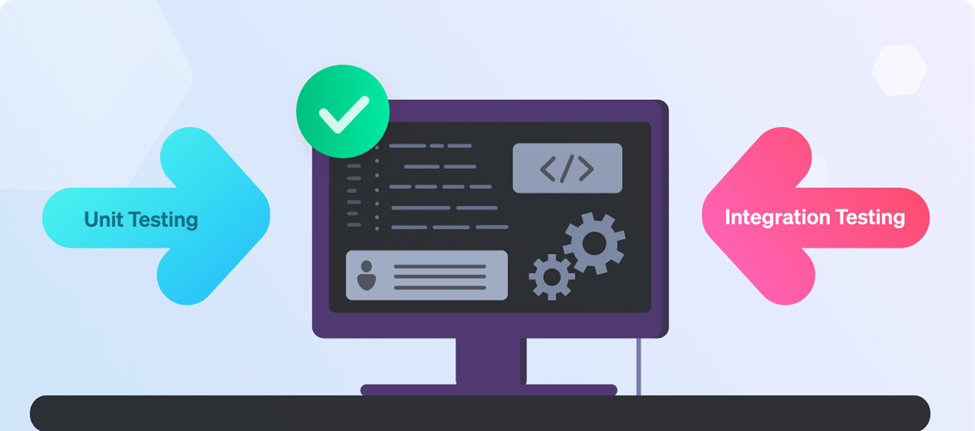
Once you feel confident with the foundations, you can investigate advanced methods to raise your test performance.
Testing Edge Cases and Exceptions
Edge case testing guarantees your code gracefully manages unanticipated inputs. For example:
[Fact] public void Divide_ByZero_ShouldThrowException() { // Arrange var calculator = new Calculator(); // Act & Assert Assert.Throws<DivideByZeroException>(() => calculator.Divide(10, 0)); } |
Edge cases are a crucial component of your testing approach since they often reveal hidden flaws not found by conventional tests.
Automating Tests with CI/CD Pipelines
Including unit tests into your CI/CD system guarantees that every commit tests your code automatically. Tools such as GitHub Actions and Azure DevOps simplify this process, lowering the risk of bug introduction into production.
Example YAML configuration for GitHub Actions:
name: .NET Tests on: push: branches: – main jobs: build: runs-on: ubuntu-latest steps: – name: Checkout code uses: actions/checkout@v2 – name: Setup .NET uses: actions/setup-dotnet@v1 with: dotnet-version: ‘6.0’ – name: Restore dependencies run: dotnet restore – name: Run tests run: dotnet test |
Code Coverage Analysis
Coverlet and ReportGenerator are two tools that will let you find out how much of your code tests cover. Measurements of code coverage give insightful information that guarantees you are not lacking important components of your application. Though you should aim for at least 80% coverage, concentrate more on addressing fundamental business logic.
Conclusion
For .NET developers hoping to produce excellent software, unit testing is a must-do habit. Early bug discovery, refactoring simplification, and enhanced teamwork help to build maintainable code from the unit tests. From organizing your surroundings using xUnit or NUnit to create challenging and advanced tests, the path to become proficient in unit testing is well worth the work.
Tests should be included into CI/CD pipelines and used tools like Moq will help to guarantee that your applications stay strong and dependable as they expand. Start small, concentrate on creating meaningful tests, and see how your process of development turns around.
Very informative
Nice Article…
Nice
The article provides valuable insights into .NET. Adding a FAQ section could address common reader questions.
It’s a good overview of the topic. Including more real-world applications could make it more impactful.
The writing style is clear and concise. Breaking down complex topics further would help beginners.
The article is structured well for learning. Including interview questions on .NET could appeal to job-seeking readers.
The content is accurate and to the point. A section highlighting .NET certifications or learning resources would be beneficial.
A useful resource for understanding .NET. Adding links to online communities or forums for further learning would make it more engaging.
A solid introduction to the topic. Addressing common challenges and solutions in .NET development could make it more insightful.
The examples are relevant and useful. More detailed explanations or tutorials could further enhance learning.
A great introduction to .NET technologies. Including a section on troubleshooting common issues could add value.
The content is well-organized and easy to follow. Adding a “Key Takeaways” section at the end would summarize the main points nicely.